Getting Started
C#
Please refer to the C# documentation.
C++
Installation
Proceed to the Bifrost pipeline on Azure DevOps, and apply a filter to display a specific release branch version as shown below image.
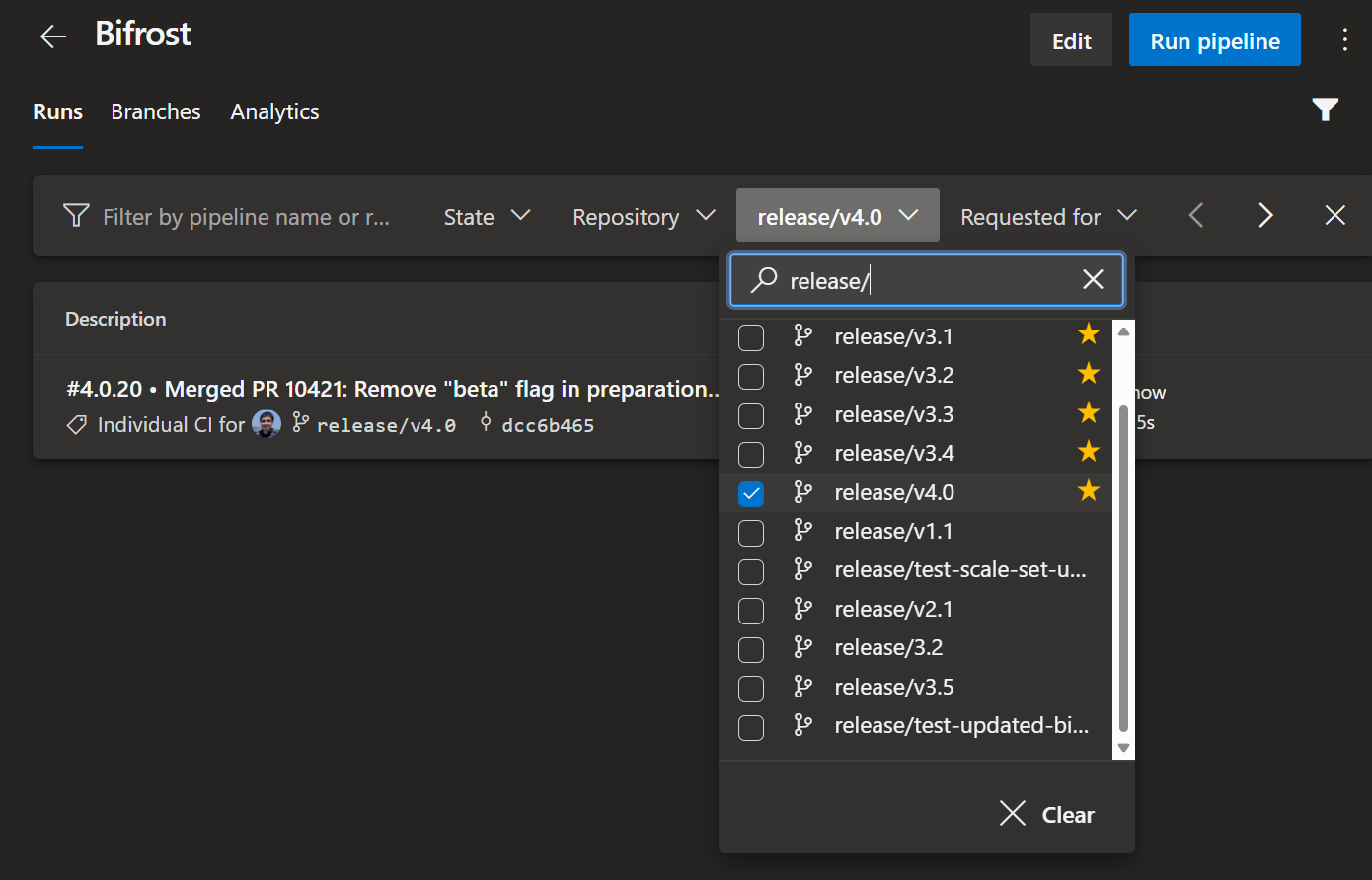
Go to the last succeeded pipeline and navigate to the published artifacts by selecting the highlighted area shown in the image below.
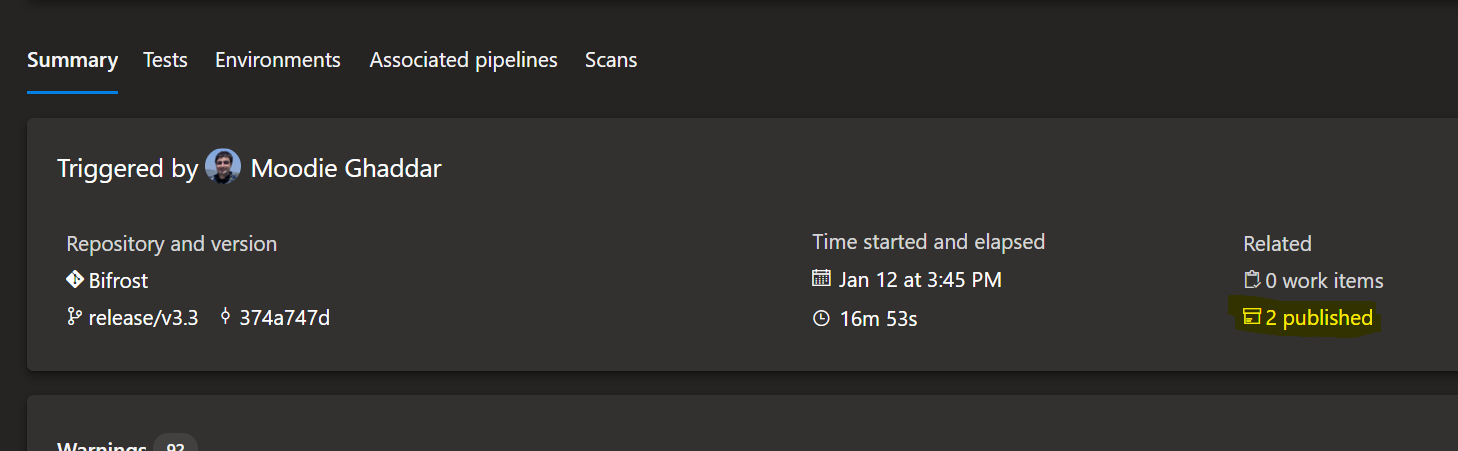
All C++ related artifacts are found under the C++/bin folder.
Infrastructure.lib: Contains the core implementation of Bifrost.
Serializer.JSON.lib: Contains the nlohmann JSON Serializer protocol implementation for the Bifrost serializer layer.
Transport.lib: Contains a Transmission Control Protocol (TCP) implementation for the Bifrost transport layer.
The subfolders within Libraries correspond to platform and version of Visual Studios used and can be excluded/included as needed within the title.
Alternatively, follow the instructions on the repository read me file to create the libraries and necessary files locally.
To start integrating Bifrost into a game project, proceed to the game integration page.
Server Interface
Bifrost servers starts listening for incoming connections after calling Start()
or StartAsync()
method. Non-immediate requests must be manually processed by calling the ProcessRequests(int numberOfRequests)
or ProcessRequestsAsync
method to allow control of the execution thread and amount of requests to process in a single frame.
The server will always send all responses in chunks to enable streaming data. The maximum chunk size may be configured when constructing the server. For streaming hooks, the server reuses a buffer equal to the maximum chunk size to avoid large memory allocations for large responses.
The Server
implementation uses a single queue for all non-immediate requests and another single queue for all responses.
Use the SendEvent(const std::string& name, std::unique_ptr<IBifrostEvent> data = nullptr)
method to send an event to all connected Bifrost clients. A BifrostEvent
can be used to construct a IBifrostEvent
. It expects two template parameters, the message type to send and the serializer type to use to encode the event. The default serializer implementations in Bifrost has a helper method to make this event easier. Assuming the JSON serializer:
// Assumes "Serializer/BifrostJsonEvent.h" is included
OnPlayerDied onPlayerDied;
onPlayerDied.playerIndex = 1;
server.SendEvent("OnPlayerDied", MakeBifrostJsonEvent(std::move(onPlayerDied)));
The C# Bifrost client will receive an event with the name “OnPlayerDied” and the attached OnPlayerDied
data.